When working with DevExpress’s ASPxTreeList control, developers often need to implement single-selection functionality. This comprehensive guide will explain how to configure ASPxTreeList only one value selectable settings and explore best practices for optimal implementation.
Understanding ASPxTreeList Selection Modes
The ASPxTreeList control offers powerful hierarchical data presentation capabilities. By default, it allows multiple selections, but many applications require restricting users to selecting only one value at a time. This functionality is particularly useful in scenarios where maintaining data integrity and preventing conflicting selections is crucial.
Key Configuration Steps for Single Selection
To make ASPxTreeList only one value selectable, you’ll need to modify several properties and potentially implement custom logic. Here’s a detailed breakdown of the essential configuration steps:
csharpCopyprotected void Page_Load(object sender, EventArgs e)
{
ASPxTreeList treeList = new ASPxTreeList();
treeList.Settings.SelectionMode = TreeListSelectionMode.Single;
treeList.SettingsBehavior.ProcessSelectionClickMode = ProcessSelectionClickMode.Single;
}
Common Use Cases and Benefits
Making ASPxTreeList only one value selectable provides several advantages in different scenarios:
- Primary Applications:
- Organizational hierarchy displays
- Category selection interfaces
- Department structure visualization
- Document management systems
- Menu configuration tools
- Key Benefits:
- Improved user experience
- Reduced data inconsistencies
- Simplified data processing
- Clear visual hierarchy
- Enhanced form validation
Advanced Configuration Options
When implementing ASPxTreeList only one value selectable functionality, you can enhance the basic setup with additional features:
javascriptCopyfunction OnSelectionChanged(s, e) {
var selectedKeys = s.GetSelectedNodeKeys();
if (selectedKeys.length > 1) {
s.UnselectAll();
s.SelectNode(selectedKeys[selectedKeys.length - 1]);
}
}
Client-Side Implementation Considerations
Client-side handling plays a crucial role in ensuring smooth operation of the ASPxTreeList single selection feature. Consider these important aspects when implementing the functionality:
javascriptCopytreelist.SettingsSelection.Enabled = true;
treelist.SettingsSelection.SelectionMode = TreeListSelectionMode.Single;
treelist.ClientSideEvents.SelectionChanged = "OnSelectionChanged";
Server-Side Processing and Validation
Proper server-side handling ensures data integrity when working with ASPxTreeList only one value selectable configurations. Implement robust validation and processing logic to maintain consistency:
csharpCopyprotected void TreeList_SelectionChanged(object sender, EventArgs e)
{
ASPxTreeList treeList = sender as ASPxTreeList;
List<object> selectedNodes = treeList.GetSelectedNodes().ToList();
if (selectedNodes.Count > 1)
{
// Maintain single selection
treeList.UnselectAll();
treeList.SelectNode(selectedNodes[0]);
}
}
Performance Optimization Tips
Ensuring optimal performance while maintaining ASPxTreeList only one value selectable functionality requires attention to several key areas:
- Implement efficient data loading mechanisms
- Optimize client-side event handling
- Minimize server roundtrips
- Use appropriate caching strategies
- Handle large datasets effectively
Troubleshooting Common Issues
When implementing ASPxTreeList only one value selectable features, developers might encounter various challenges. Here are solutions to common problems:
- Selection persistence issues across postbacks
- Conflicts with other control events
- Performance degradation with large datasets
- Browser compatibility concerns
- Integration challenges with existing code
Integration with Other Controls
ASPxTreeList only one value selectable functionality often needs to work seamlessly with other controls and components in your application. Consider these integration patterns:
csharpCopyprotected void SynchronizeControls()
{
var selectedNode = treeList.GetSelectedNodes().FirstOrDefault();
if (selectedNode != null)
{
// Update related controls
otherControl.Value = selectedNode.Key.ToString();
}
}
Best Practices and Recommendations
To ensure successful implementation of ASPxTreeList only one value selectable features, follow these recommended practices:
- Always implement proper error handling
- Document custom implementations thoroughly
- Consider accessibility requirements
- Maintain consistent user experience
- Plan for scalability from the start
Conclusion
Implementing ASPxTreeList only one value selectable functionality requires careful consideration of various aspects, from basic configuration to advanced customization. By following the guidelines and best practices outlined in this article, developers can create robust and user-friendly tree list implementations that maintain data integrity through single-selection functionality.
Remember to test thoroughly across different scenarios and user interactions to ensure your implementation meets all requirements while maintaining optimal performance. With proper configuration and attention to detail, the ASPxTreeList control can provide a powerful and intuitive single-selection interface for your applications.
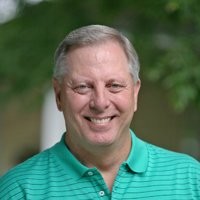
Mark Thompson, a seasoned pest controller, is renowned for his expertise in keeping homes and businesses free from unwanted intruders. With a passion for environmental sustainability and a deep understanding of pest behavior, Mark has become a trusted authority in the industry.